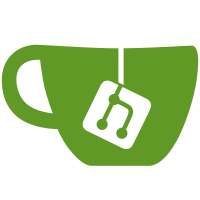
Now that the toolchain supports multiple mirrors, we can have more robust defaults: try ziglang.org first, then dl.jakstys.lt when that one removes the build we rely on. This will make builds less prone to inevitable failures of my home server.
190 lines
6.2 KiB
Python
190 lines
6.2 KiB
Python
load("@bazel_tools//tools/build_defs/repo:http.bzl", "http_archive")
|
|
load("@bazel_tools//tools/build_defs/repo:utils.bzl", "read_user_netrc", "use_netrc")
|
|
load("@bazel-zig-cc//toolchain/private:defs.bzl", "DEFAULT_INCLUDE_DIRECTORIES", "ZIG_TOOL_PATH", "target_structs")
|
|
|
|
_fcntl_map = """
|
|
GLIBC_2.2.5 {
|
|
fcntl;
|
|
};
|
|
"""
|
|
_fcntl_h = """
|
|
#ifdef __ASSEMBLER__
|
|
.symver fcntl64, fcntl@GLIBC_2.2.5
|
|
#else
|
|
__asm__(".symver fcntl64, fcntl@GLIBC_2.2.5");
|
|
#endif
|
|
"""
|
|
|
|
# Official recommended version. Should use this when we have a usable release.
|
|
URL_FORMAT_RELEASE = "https://ziglang.org/download/{version}/zig-{host_platform}-{version}.tar.xz"
|
|
|
|
# Caution: nightly releases are purged from ziglang.org after ~90 days. A real
|
|
# solution would be to allow the downstream project specify their own mirrors.
|
|
# This is explained in
|
|
# https://sr.ht/~motiejus/bazel-zig-cc/#alternative-download-urls and is
|
|
# awaiting my attention or your contribution.
|
|
URL_FORMAT_NIGHTLY = "https://ziglang.org/builds/zig-{host_platform}-{version}.tar.xz"
|
|
|
|
# Author's mirror that doesn't purge the nightlies so aggressively. I will be
|
|
# cleaning those up manually only after the artifacts are not in use for many
|
|
# months in bazel-zig-cc. dl.jakstys.lt is a small x86_64 server with an NVMe
|
|
# drive sitting in my home closet on a 1GB/s symmetric residential connection,
|
|
# which, as of writing, has been quite reliable.
|
|
URL_FORMAT_JAKSTYS = "https://dl.jakstys.lt/zig/zig-{host_platform}-{version}.tar.xz"
|
|
|
|
_VERSION = "0.10.0-dev.2252+a4369918b"
|
|
|
|
_HOST_PLATFORM_SHA256 = {
|
|
"linux-aarch64": "a88d646f881ae39bde4631e2b48d986a90878f6fadb1a588c8e297a52ac1b606",
|
|
"linux-x86_64": "1d3c3769eba85a4334c93a3cfa35ad0ef914dd8cf9fd502802004c6908f5370c",
|
|
"macos-aarch64": "ab46e7499e5bd7b6d6ff2ac331e1a4aa875a01b270dc40306bc29dbaf216fccf",
|
|
"macos-x86_64": "fb213f996bcab805839e401292c42a92b63cd97deb1631e31bd61f534b7f6b1c",
|
|
}
|
|
|
|
def toolchains(
|
|
version = _VERSION,
|
|
url_formats = [URL_FORMAT_NIGHTLY, URL_FORMAT_JAKSTYS],
|
|
host_platform_sha256 = _HOST_PLATFORM_SHA256):
|
|
"""
|
|
Download zig toolchain and declare bazel toolchains.
|
|
The platforms are not registered automatically, that should be done by
|
|
the user with register_toolchains() in the WORKSPACE file. See README
|
|
for possible choices.
|
|
"""
|
|
zig_repository(
|
|
name = "zig_sdk",
|
|
version = version,
|
|
url_formats = url_formats,
|
|
host_platform_sha256 = host_platform_sha256,
|
|
host_platform_include_root = {
|
|
"linux-aarch64": "lib/zig/",
|
|
"linux-x86_64": "lib/",
|
|
"macos-aarch64": "lib/zig/",
|
|
"macos-x86_64": "lib/zig/",
|
|
},
|
|
)
|
|
|
|
ZIG_TOOL_WRAPPER = """#!/bin/bash
|
|
set -e
|
|
|
|
if [[ -n "$TMPDIR" ]]; then
|
|
_cache_prefix=$TMPDIR
|
|
else
|
|
_cache_prefix="$HOME/.cache"
|
|
if [[ "$(uname)" = Darwin ]]; then
|
|
_cache_prefix="$HOME/Library/Caches"
|
|
fi
|
|
fi
|
|
export ZIG_LOCAL_CACHE_DIR="$_cache_prefix/bazel-zig-cc"
|
|
export ZIG_GLOBAL_CACHE_DIR=$ZIG_LOCAL_CACHE_DIR
|
|
|
|
exec "{zig}" "{zig_tool}" "$@"
|
|
"""
|
|
|
|
_ZIG_TOOLS = [
|
|
"c++",
|
|
"cc",
|
|
"ar",
|
|
"ld.lld", # ELF
|
|
"ld64.lld", # Mach-O
|
|
"lld-link", # COFF
|
|
"wasm-ld", # WebAssembly
|
|
]
|
|
|
|
def _quote(s):
|
|
return "'" + s.replace("'", "'\\''") + "'"
|
|
|
|
def _zig_repository_impl(repository_ctx):
|
|
arch = repository_ctx.os.arch
|
|
if arch == "amd64":
|
|
arch = "x86_64"
|
|
|
|
os = repository_ctx.os.name.lower()
|
|
if os.startswith("mac os"):
|
|
os = "macos"
|
|
|
|
host_platform = "{}-{}".format(os, arch)
|
|
|
|
zig_include_root = repository_ctx.attr.host_platform_include_root[host_platform]
|
|
zig_sha256 = repository_ctx.attr.host_platform_sha256[host_platform]
|
|
format_vars = {
|
|
"version": repository_ctx.attr.version,
|
|
"host_platform": host_platform,
|
|
}
|
|
|
|
urls = [uf.format(**format_vars) for uf in repository_ctx.attr.url_formats]
|
|
repository_ctx.download_and_extract(
|
|
auth = use_netrc(read_user_netrc(repository_ctx), urls, {}),
|
|
url = urls,
|
|
stripPrefix = "zig-{host_platform}-{version}/".format(**format_vars),
|
|
sha256 = zig_sha256,
|
|
)
|
|
|
|
for zig_tool in _ZIG_TOOLS:
|
|
repository_ctx.file(
|
|
ZIG_TOOL_PATH.format(zig_tool = zig_tool),
|
|
ZIG_TOOL_WRAPPER.format(
|
|
zig = str(repository_ctx.path("zig")),
|
|
zig_tool = zig_tool,
|
|
),
|
|
)
|
|
|
|
repository_ctx.file(
|
|
"glibc-hacks/fcntl.map",
|
|
content = _fcntl_map,
|
|
)
|
|
repository_ctx.file(
|
|
"glibc-hacks/glibchack-fcntl.h",
|
|
content = _fcntl_h,
|
|
)
|
|
|
|
for dest, src in {
|
|
"platform/BUILD": "//toolchain/platform:BUILD",
|
|
"toolchain/BUILD": "//toolchain/toolchain:BUILD",
|
|
"libc/BUILD": "//toolchain/libc:BUILD",
|
|
"libc_aware/platform/BUILD": "//toolchain/libc_aware/platform:BUILD",
|
|
"libc_aware/toolchain/BUILD": "//toolchain/libc_aware/toolchain:BUILD",
|
|
}.items():
|
|
repository_ctx.symlink(Label(src), dest)
|
|
|
|
for dest, src in {
|
|
"BUILD": "//toolchain:BUILD.sdk.bazel",
|
|
"private/BUILD": "//toolchain/private:BUILD.sdk.bazel",
|
|
}.items():
|
|
repository_ctx.template(
|
|
dest,
|
|
Label(src),
|
|
executable = False,
|
|
substitutions = {
|
|
"{absolute_path}": _quote(str(repository_ctx.path(""))),
|
|
"{zig_include_root}": _quote(zig_include_root),
|
|
},
|
|
)
|
|
|
|
zig_repository = repository_rule(
|
|
attrs = {
|
|
"version": attr.string(),
|
|
"host_platform_sha256": attr.string_dict(),
|
|
"url_formats": attr.string_list(allow_empty = False),
|
|
"host_platform_include_root": attr.string_dict(),
|
|
},
|
|
implementation = _zig_repository_impl,
|
|
)
|
|
|
|
def filegroup(name, **kwargs):
|
|
native.filegroup(name = name, **kwargs)
|
|
return ":" + name
|
|
|
|
def declare_files(zig_include_root):
|
|
filegroup(name = "empty")
|
|
native.exports_files(["zig"], visibility = ["//visibility:public"])
|
|
filegroup(name = "lib/std", srcs = native.glob(["lib/std/**"]))
|
|
|
|
lazy_filegroups = {}
|
|
|
|
for target_config in target_structs():
|
|
for d in DEFAULT_INCLUDE_DIRECTORIES + target_config.includes:
|
|
d = zig_include_root + d
|
|
if d not in lazy_filegroups:
|
|
lazy_filegroups[d] = filegroup(name = d, srcs = native.glob([d + "/**"]))
|